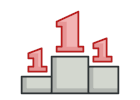
TypeScript 单例模式讲解和代码示例
单例是一种创建型设计模式, 让你能够保证一个类只有一个实例, 并提供一个访问该实例的全局节点。
单例拥有与全局变量相同的优缺点。 尽管它们非常有用, 但却会破坏代码的模块化特性。
在某些其他上下文中, 你不能使用依赖于单例的类。 你也将必须使用单例类。 绝大多数情况下, 该限制会在创建单元测试时出现。
复杂度:
流行度:
使用示例: 许多开发者将单例模式视为一种反模式。 因此它在 TypeScript 代码中的使用频率正在逐步减少。
识别方法: 单例可以通过返回相同缓存对象的静态构建方法来识别。
概念示例
本例说明了单例设计模式的结构并重点回答了下面的问题:
- 它由哪些类组成?
- 这些类扮演了哪些角色?
- 模式中的各个元素会以何种方式相互关联?
index.ts: 概念示例
/**
* The Singleton class defines an `instance` getter, that lets clients access
* the unique singleton instance.
*/
class Singleton {
static #instance: Singleton;
/**
* The Singleton's constructor should always be private to prevent direct
* construction calls with the `new` operator.
*/
private constructor() { }
/**
* The static getter that controls access to the singleton instance.
*
* This implementation allows you to extend the Singleton class while
* keeping just one instance of each subclass around.
*/
public static get instance(): Singleton {
if (!Singleton.#instance) {
Singleton.#instance = new Singleton();
}
return Singleton.#instance;
}
/**
* Finally, any singleton can define some business logic, which can be
* executed on its instance.
*/
public someBusinessLogic() {
// ...
}
}
/**
* The client code.
*/
function clientCode() {
const s1 = Singleton.instance;
const s2 = Singleton.instance;
if (s1 === s2) {
console.log(
'Singleton works, both variables contain the same instance.'
);
} else {
console.log('Singleton failed, variables contain different instances.');
}
}
clientCode();
Output.txt: 执行结果
Singleton works, both variables contain the same instance.