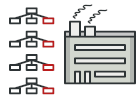
Абстрактная фабрика на Swift
Абстрактная фабрика — это порождающий паттерн проектирования, который решает проблему создания целых семейств связанных продуктов, без указания конкретных классов продуктов.
Абстрактная фабрика задаёт интерфейс создания всех доступных типов продуктов, а каждая конкретная реализация фабрики порождает продукты одной из вариаций. Клиентский код вызывает методы фабрики для получения продуктов, вместо самостоятельного создания с помощью оператора new
. При этом фабрика сама следит за тем, чтобы создать продукт нужной вариации.
Сложность:
Популярность:
Применимость: Паттерн можно часто встретить в Swift-коде, особенно там, где требуется создание семейств продуктов (например, внутри фреймворков).
Признаки применения паттерна: Паттерн можно определить по методам, возвращающим фабрику, которая, в свою очередь, используется для создания конкретных продуктов, возвращая их через абстрактные типы или интерфейсы.
Концептуальный пример
Этот пример показывает структуру паттерна Абстрактная фабрика, а именно — из каких классов он состоит, какие роли эти классы выполняют и как они взаимодействуют друг с другом.
После ознакомления со структурой, вам будет легче воспринимать второй пример, который рассматривает реальный случай использования паттерна в мире Swift.
Example.swift: Пример структуры паттерна
import XCTest
/// Интерфейс Абстрактной Фабрики объявляет набор методов, которые возвращают
/// различные абстрактные продукты. Эти продукты называются семейством и связаны
/// темой или концепцией высокого уровня. Продукты одного семейства обычно могут
/// взаимодействовать между собой. Семейство продуктов может иметь несколько
/// вариаций, но продукты одной вариации несовместимы с продуктами другой.
protocol AbstractFactory {
func createProductA() -> AbstractProductA
func createProductB() -> AbstractProductB
}
/// Конкретная Фабрика производит семейство продуктов одной вариации. Фабрика
/// гарантирует совместимость полученных продуктов. Обратите внимание, что
/// сигнатуры методов Конкретной Фабрики возвращают абстрактный продукт, в то
/// время как внутри метода создается экземпляр конкретного продукта.
class ConcreteFactory1: AbstractFactory {
func createProductA() -> AbstractProductA {
return ConcreteProductA1()
}
func createProductB() -> AbstractProductB {
return ConcreteProductB1()
}
}
/// Каждая Конкретная Фабрика имеет соответствующую вариацию продукта.
class ConcreteFactory2: AbstractFactory {
func createProductA() -> AbstractProductA {
return ConcreteProductA2()
}
func createProductB() -> AbstractProductB {
return ConcreteProductB2()
}
}
/// Каждый отдельный продукт семейства продуктов должен иметь базовый интерфейс.
/// Все вариации продукта должны реализовывать этот интерфейс.
protocol AbstractProductA {
func usefulFunctionA() -> String
}
/// Конкретные продукты создаются соответствующими Конкретными Фабриками.
class ConcreteProductA1: AbstractProductA {
func usefulFunctionA() -> String {
return "The result of the product A1."
}
}
class ConcreteProductA2: AbstractProductA {
func usefulFunctionA() -> String {
return "The result of the product A2."
}
}
/// Базовый интерфейс другого продукта. Все продукты могут взаимодействовать
/// друг с другом, но правильное взаимодействие возможно только между продуктами
/// одной и той же конкретной вариации.
protocol AbstractProductB {
/// Продукт B способен работать самостоятельно...
func usefulFunctionB() -> String
/// ...а также взаимодействовать с Продуктами A той же вариации.
///
/// Абстрактная Фабрика гарантирует, что все продукты, которые она создает,
/// имеют одинаковую вариацию и, следовательно, совместимы.
func anotherUsefulFunctionB(collaborator: AbstractProductA) -> String
}
/// Конкретные Продукты создаются соответствующими Конкретными Фабриками.
class ConcreteProductB1: AbstractProductB {
func usefulFunctionB() -> String {
return "The result of the product B1."
}
/// Продукт B1 может корректно работать только с Продуктом A1. Тем не менее,
/// он принимает любой экземпляр Абстрактного Продукта А в качестве
/// аргумента.
func anotherUsefulFunctionB(collaborator: AbstractProductA) -> String {
let result = collaborator.usefulFunctionA()
return "The result of the B1 collaborating with the (\(result))"
}
}
class ConcreteProductB2: AbstractProductB {
func usefulFunctionB() -> String {
return "The result of the product B2."
}
/// Продукт B2 может корректно работать только с Продуктом A2. Тем не менее,
/// он принимает любой экземпляр Абстрактного Продукта А в качестве
/// аргумента.
func anotherUsefulFunctionB(collaborator: AbstractProductA) -> String {
let result = collaborator.usefulFunctionA()
return "The result of the B2 collaborating with the (\(result))"
}
}
/// Клиентский код работает с фабриками и продуктами только через абстрактные
/// типы: Абстрактная Фабрика и Абстрактный Продукт. Это позволяет передавать
/// любой подкласс фабрики или продукта клиентскому коду, не нарушая его.
class Client {
// ...
static func someClientCode(factory: AbstractFactory) {
let productA = factory.createProductA()
let productB = factory.createProductB()
print(productB.usefulFunctionB())
print(productB.anotherUsefulFunctionB(collaborator: productA))
}
// ...
}
/// Давайте посмотрим как всё это будет работать.
class AbstractFactoryConceptual: XCTestCase {
func testAbstractFactoryConceptual() {
/// Клиентский код может работать с любым конкретным классом фабрики.
print("Client: Testing client code with the first factory type:")
Client.someClientCode(factory: ConcreteFactory1())
print("Client: Testing the same client code with the second factory type:")
Client.someClientCode(factory: ConcreteFactory2())
}
}
Output.txt: Результат выполнения
Client: Testing client code with the first factory type:
The result of the product B1.
The result of the B1 collaborating with the (The result of the product A1.)
Client: Testing the same client code with the second factory type:
The result of the product B2.
The result of the B2 collaborating with the (The result of the product A2.)
Пример из реальной жизни
Example.swift: Пример из реальной жизни
import Foundation
import UIKit
import XCTest
enum AuthType {
case login
case signUp
}
protocol AuthViewFactory {
static func authView(for type: AuthType) -> AuthView
static func authController(for type: AuthType) -> AuthViewController
}
class StudentAuthViewFactory: AuthViewFactory {
static func authView(for type: AuthType) -> AuthView {
print("Student View has been created")
switch type {
case .login: return StudentLoginView()
case .signUp: return StudentSignUpView()
}
}
static func authController(for type: AuthType) -> AuthViewController {
let controller = StudentAuthViewController(contentView: authView(for: type))
print("Student View Controller has been created")
return controller
}
}
class TeacherAuthViewFactory: AuthViewFactory {
static func authView(for type: AuthType) -> AuthView {
print("Teacher View has been created")
switch type {
case .login: return TeacherLoginView()
case .signUp: return TeacherSignUpView()
}
}
static func authController(for type: AuthType) -> AuthViewController {
let controller = TeacherAuthViewController(contentView: authView(for: type))
print("Teacher View Controller has been created")
return controller
}
}
protocol AuthView {
typealias AuthAction = (AuthType) -> ()
var contentView: UIView { get }
var authHandler: AuthAction? { get set }
var description: String { get }
}
class StudentSignUpView: UIView, AuthView {
private class StudentSignUpContentView: UIView {
/// This view contains a number of features available only during a
/// STUDENT authorization.
}
var contentView: UIView = StudentSignUpContentView()
/// The handler will be connected for actions of buttons of this view.
var authHandler: AuthView.AuthAction?
override var description: String {
return "Student-SignUp-View"
}
}
class StudentLoginView: UIView, AuthView {
private let emailField = UITextField()
private let passwordField = UITextField()
private let signUpButton = UIButton()
var contentView: UIView {
return self
}
/// The handler will be connected for actions of buttons of this view.
var authHandler: AuthView.AuthAction?
override var description: String {
return "Student-Login-View"
}
}
class TeacherSignUpView: UIView, AuthView {
class TeacherSignUpContentView: UIView {
/// This view contains a number of features available only during a
/// TEACHER authorization.
}
var contentView: UIView = TeacherSignUpContentView()
/// The handler will be connected for actions of buttons of this view.
var authHandler: AuthView.AuthAction?
override var description: String {
return "Teacher-SignUp-View"
}
}
class TeacherLoginView: UIView, AuthView {
private let emailField = UITextField()
private let passwordField = UITextField()
private let loginButton = UIButton()
private let forgotPasswordButton = UIButton()
var contentView: UIView {
return self
}
/// The handler will be connected for actions of buttons of this view.
var authHandler: AuthView.AuthAction?
override var description: String {
return "Teacher-Login-View"
}
}
class AuthViewController: UIViewController {
fileprivate var contentView: AuthView
init(contentView: AuthView) {
self.contentView = contentView
super.init(nibName: nil, bundle: nil)
}
required convenience init?(coder aDecoder: NSCoder) {
return nil
}
}
class StudentAuthViewController: AuthViewController {
/// Student-oriented features
}
class TeacherAuthViewController: AuthViewController {
/// Teacher-oriented features
}
private class ClientCode {
private var currentController: AuthViewController?
private lazy var navigationController: UINavigationController = {
guard let vc = currentController else { return UINavigationController() }
return UINavigationController(rootViewController: vc)
}()
private let factoryType: AuthViewFactory.Type
init(factoryType: AuthViewFactory.Type) {
self.factoryType = factoryType
}
/// MARK: - Presentation
func presentLogin() {
let controller = factoryType.authController(for: .login)
navigationController.pushViewController(controller, animated: true)
}
func presentSignUp() {
let controller = factoryType.authController(for: .signUp)
navigationController.pushViewController(controller, animated: true)
}
/// Other methods...
}
class AbstractFactoryRealWorld: XCTestCase {
func testFactoryMethodRealWorld() {
#if teacherMode
let clientCode = ClientCode(factoryType: TeacherAuthViewFactory.self)
#else
let clientCode = ClientCode(factoryType: StudentAuthViewFactory.self)
#endif
/// Present LogIn flow
clientCode.presentLogin()
print("Login screen has been presented")
/// Present SignUp flow
clientCode.presentSignUp()
print("Sign up screen has been presented")
}
}
Output.txt: Результат выполнения
Teacher View has been created
Teacher View Controller has been created
Login screen has been presented
Teacher View has been created
Teacher View Controller has been created
Sign up screen has been presented