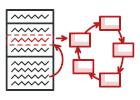
Python 状态模式讲解和代码示例
状态是一种行为设计模式, 让你能在一个对象的内部状态变化时改变其行为。
该模式将与状态相关的行为抽取到独立的状态类中, 让原对象将工作委派给这些类的实例, 而不是自行进行处理。
复杂度:
流行度:
使用示例: 在 Python 语言中, 状态模式通常被用于将基于 switch
语句的大型状态机转换为对象。
识别方法: 状态模式可通过受外部控制且能根据对象状态改变行为的方法来识别。
概念示例
本例说明了状态设计模式的结构并重点回答了下面的问题:
- 它由哪些类组成?
- 这些类扮演了哪些角色?
- 模式中的各个元素会以何种方式相互关联?
main.py: 概念示例
from __future__ import annotations
from abc import ABC, abstractmethod
class Context:
"""
The Context defines the interface of interest to clients. It also maintains
a reference to an instance of a State subclass, which represents the current
state of the Context.
"""
_state = None
"""
A reference to the current state of the Context.
"""
def __init__(self, state: State) -> None:
self.transition_to(state)
def transition_to(self, state: State):
"""
The Context allows changing the State object at runtime.
"""
print(f"Context: Transition to {type(state).__name__}")
self._state = state
self._state.context = self
"""
The Context delegates part of its behavior to the current State object.
"""
def request1(self):
self._state.handle1()
def request2(self):
self._state.handle2()
class State(ABC):
"""
The base State class declares methods that all Concrete State should
implement and also provides a backreference to the Context object,
associated with the State. This backreference can be used by States to
transition the Context to another State.
"""
@property
def context(self) -> Context:
return self._context
@context.setter
def context(self, context: Context) -> None:
self._context = context
@abstractmethod
def handle1(self) -> None:
pass
@abstractmethod
def handle2(self) -> None:
pass
"""
Concrete States implement various behaviors, associated with a state of the
Context.
"""
class ConcreteStateA(State):
def handle1(self) -> None:
print("ConcreteStateA handles request1.")
print("ConcreteStateA wants to change the state of the context.")
self.context.transition_to(ConcreteStateB())
def handle2(self) -> None:
print("ConcreteStateA handles request2.")
class ConcreteStateB(State):
def handle1(self) -> None:
print("ConcreteStateB handles request1.")
def handle2(self) -> None:
print("ConcreteStateB handles request2.")
print("ConcreteStateB wants to change the state of the context.")
self.context.transition_to(ConcreteStateA())
if __name__ == "__main__":
# The client code.
context = Context(ConcreteStateA())
context.request1()
context.request2()
Output.txt: 执行结果
Context: Transition to ConcreteStateA
ConcreteStateA handles request1.
ConcreteStateA wants to change the state of the context.
Context: Transition to ConcreteStateB
ConcreteStateB handles request2.
ConcreteStateB wants to change the state of the context.
Context: Transition to ConcreteStateA